Understanding the Importance of Components in React
When it comes to React, components are the building blocks that everything revolves around. They form the foundation of any React project and play a crucial role in structuring and customizing your applications. In a recent tweet, Rishabh Pal highlighted the significance of components in React and how they are essential for creating dynamic and interactive user interfaces.
In React, you’ll learn how to build components from scratch, customize them to suit your needs, and compose layouts by importing components into other components. This modular approach not only makes your code more organized and maintainable but also allows you to reuse components across different parts of your application.
You may also like to watch : Who Is Kamala Harris? Biography - Parents - Husband - Sister - Career - Indian - Jamaican Heritage
By understanding the role of components in React, you can create more efficient and scalable applications that are easier to manage and update. Whether you’re a beginner learning the basics of React or an experienced developer looking to enhance your skills, mastering components is essential for building high-quality web applications.
So, the next time you’re working on a React project, remember the importance of components and how they can help you create powerful and engaging user interfaces. With components at the core of your development process, you’ll be able to build interactive web applications that delight users and enhance their overall experience.
Components are one of the foundations of React. In React, everything revolves around components. You’ll learn how to build components, how to structure and customize your React projects, and how to compose layouts by importing components into other components.@Meta
— Rishabh Pal (@rishabhpal326) July 24, 2024
You may also like to watch: Is US-NATO Prepared For A Potential Nuclear War With Russia - China And North Korea?
Components are one of the fundamental building blocks of React, a popular JavaScript library for building user interfaces. In React, everything revolves around components, which are reusable pieces of code that encapsulate a part of the user interface. In this article, we will explore the importance of components in React, how to build and customize them, and how to compose layouts by importing components into other components.
What are components in React?
In React, components are the building blocks of a UI. They are like small, reusable pieces of code that can be combined to create complex user interfaces. Components can be either class components or functional components. Class components are ES6 classes that extend from React.Component and have a render method, while functional components are just JavaScript functions that return JSX.
How can you build components in React?
To build a component in React, you can create a new JavaScript file for your component and define it as either a class component or a functional component. For example, if you want to create a simple functional component that displays a greeting message, you can write the following code:
function Greeting() {<br />
return <h1>Hello, World!</h1>;<br />
}<br />
```<br />
<br />
You can then use this component in your React application by importing it and including it in your JSX code. Components can also accept props, which are parameters that allow you to customize the behavior of the component.<br />
<br />
### How do you structure and customize your React projects?<br />
<br />
When working on a larger React project, it's important to organize your components in a structured way to make your code more maintainable and scalable. One common practice is to create a components folder where you store all your reusable components. You can further organize your components into subfolders based on their functionality.<br />
<br />
To customize your components, you can pass props to them to change their behavior or appearance. For example, you can pass a prop to a Button component to change its color or label. You can also use state to manage the internal state of a component and update it based on user interactions.<br />
<br />
### How do you compose layouts by importing components into other components?<br />
<br />
One of the key features of React is the ability to compose layouts by nesting components inside other components. This allows you to break down your UI into smaller, reusable pieces and compose them together to create complex layouts. For example, you can create a Layout component that includes a Header component, a Sidebar component, and a Main component:<br />
<br />
```jsx<br />
function Layout() {<br />
return (<br />
<div><br />
<Header /><br />
<Sidebar /><br />
<Main /><br />
</div><br />
);<br />
}<br />
```<br />
<br />
By importing and nesting components like this, you can easily create and manage the layout of your application. This compositional approach also promotes code reusability and maintainability.<br />
<br />
In conclusion, components are the foundation of React and play a crucial role in building modern web applications. By understanding how to build, structure, customize, and compose components, you can create more efficient and maintainable React projects. So, next time you start a new React project, remember to think in components and leverage their power to create amazing user interfaces.<br />
<br />
Sources:<br />
- <a href="https://reactjs.org/docs/components-and-props.html">React Documentation - Components and Props</a><br />
- <a href="https://reactpatterns.com/">React Patterns</a>
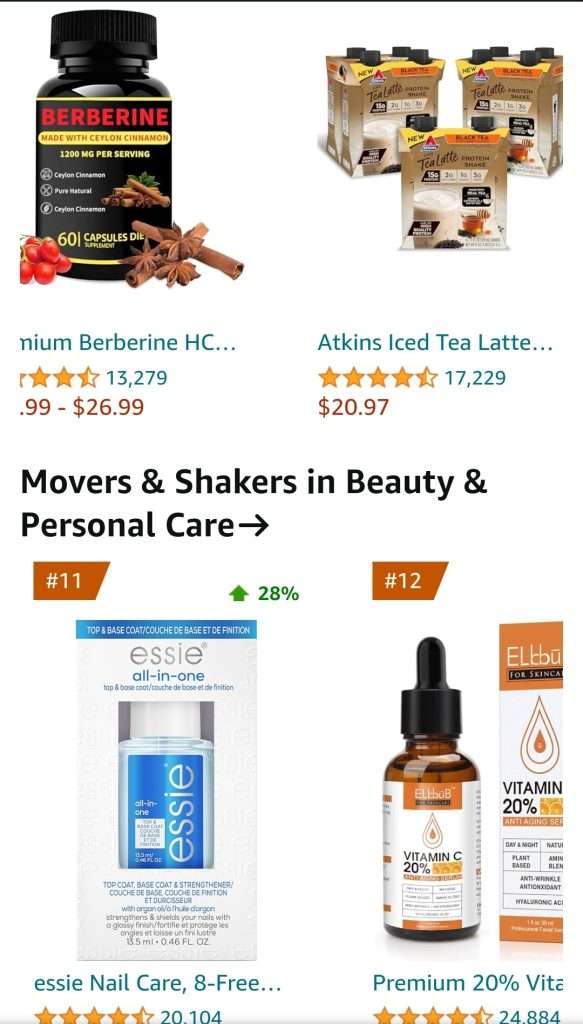
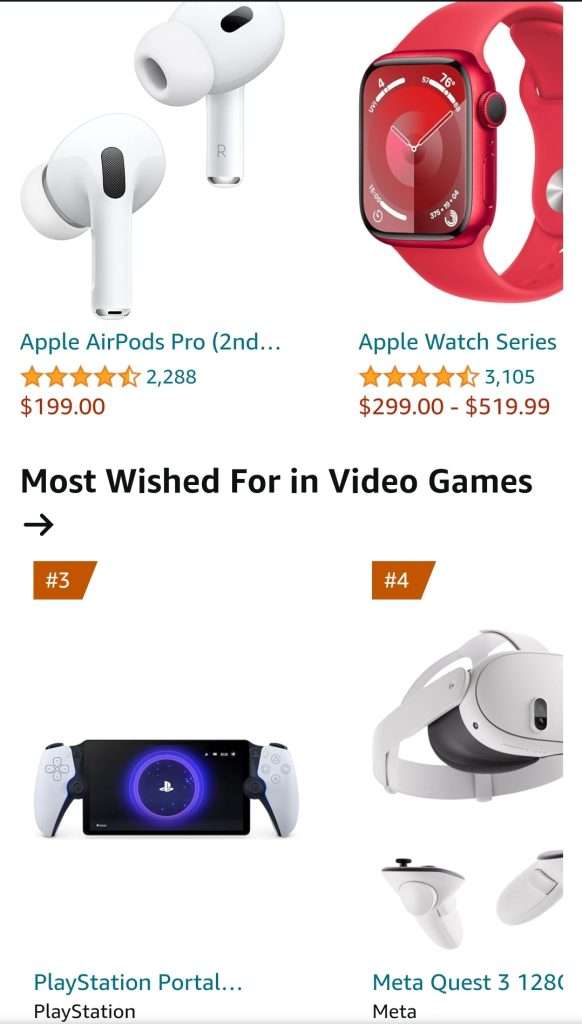
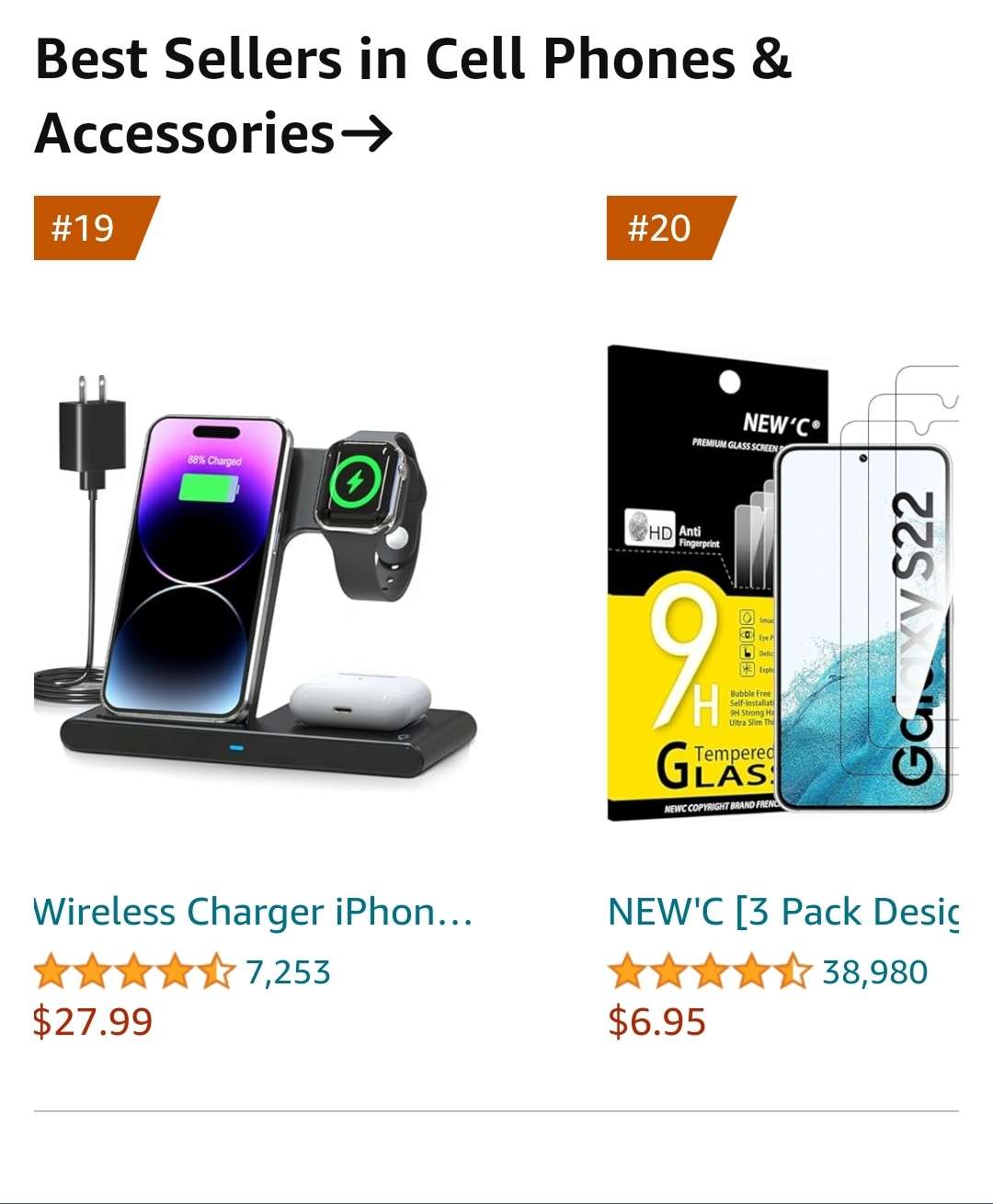